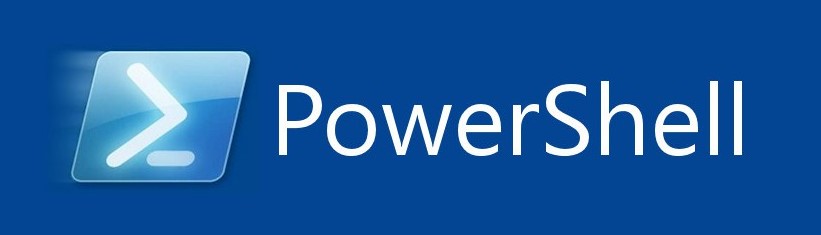
I recently found myself in a situation on a client’s device where I needed to remove an application, but this was not possible because I was not a local administrator on the device. The client allows elevation using a third party application when you need to run programs as an administrator and that allows applications to be installed, but when you try to delete an app from the Control Panel or “Apps & Features,” there is no option to use the third party application to elevate you to administrator.
After finding a few options, like using the ages old WMI client which is glacially slow, I decided to turn to my old friend PowerShell. And viola! PowerShell provides a simple and fast way to search for the correct application and then remove it. Realizing that it was possible to accidentally remove much more than I wanted, most of the script is around making sure only one app can be deleted at a time.
On to the code
To run this, open PowerShell as an Administrator and run the following:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
|
function Uninstall-App {
<#
.SYNOPSIS
Uninstalls Windows application using search to find the correct app.
.DESCRIPTION
This function uses the Filter parameter to search through Windows applications
for the targeted app. If the parameter is not provided, the user will
be prompted. The function will only offer to uninstall if the search
returns one app.
.PARAMETER Filter
A string that is used to search application based on Name or TagId.
.EXAMPLE
Uninstall-App -Filter "Node.js"
#>
[cmdletbinding()]
# String to base the search on
Param ([string]$Filter)
# End of Parameters
Process {
Clear-Host
if ($Filter -eq '' ) {
$Filter = Read-Host ("Enter the app Name or TagId to search for")
if ($Filter -eq '') {
Write-host "`r`nNo search criteria entered. Exiting." -ForegroundColor Yellow
return
}
}
write-host "`r`nSearching for '$($Filter)' ..."
$apps = Get-Package | ?{ ($_.Name -like "*$($Filter)*" -or $_.TagId -like "*$($Filter)*") -and $_.ProviderName -eq "msi" } | select Name, Version, TagId
if ($apps.Count -eq 0) {
write-host "`r`nYour search returned no results." -ForegroundColor Yellow
return
}
if ($apps.Count -gt 1) {
write-host "`r`nYour search returned more than one app, but only one app can be `r`ndeleted at a time. Please adjust the filter and try again." -ForegroundColor Yellow
write-host "HINT: Use the TagId to filter your search." -ForegroundColor Yellow
write-host "`r`nApps that match your request:" -ForegroundColor Yellow
$apps | sort-object { $_.Name } | Format-Table Name,TagId,Version -AutoSize
return
}
$apps[0] | Format-Table Name,TagId,Version -AutoSize
$confirm = Read-Host ("Confirm you want to remove the application listed above (Y/n)")
if ($confirm -eq 'y' -or $confirm -eq '') {
write-host "`r`nUninstalling ... " -ForegroundColor Green
Uninstall-Package -Name $apps[0].Name -RequiredVersion $apps[0].Version
}
else {
Write-host "Exiting."
}
}
}
Uninstall-App
|